Powershell Excel Power: 20+ Tips To Open Files On Sharepoint With Ease: Master The Art
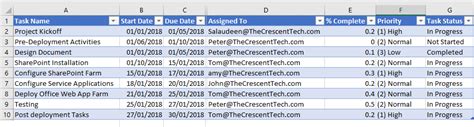
Opening files on SharePoint can be a breeze with the right PowerShell commands and techniques. In this blog post, we will explore over 20 tips and tricks to streamline your file opening process, making it more efficient and user-friendly. By the end, you'll be a master at navigating and accessing files on SharePoint with PowerShell.
Navigating SharePoint with PowerShell

PowerShell is a powerful tool that allows you to interact with SharePoint programmatically. With the right commands, you can automate tasks, manage files, and enhance your overall productivity. Let's dive into some essential tips for opening files on SharePoint effortlessly.
1. Connect to SharePoint Site

Before you can access files, you need to establish a connection to your SharePoint site. Here's how you can do it:
$siteUrl = "https://yoursharepointsite.com"
$userName = "yourusername"
$password = ConvertTo-SecureString "yourpassword" -AsPlainText -Force
$credential = New-Object System.Management.Automation.PSCredential ($userName, $password)
Connect-PnPOnline -Url $siteUrl -Credentials $credential
Replace "https://yoursharepointsite.com"
, "yourusername"
, and "yourpassword"
with your actual SharePoint site URL and credentials.
2. Get List of Files

Once connected, you can retrieve a list of files from a specific library or folder on SharePoint. This is useful when you want to display available files to the user or perform bulk operations.
$files = Get-PnPFile -Url "Shared Documents"
foreach ($file in $files) {
Write-Host $file.Name
}
The above code retrieves files from the "Shared Documents"
library and displays their names.
3. Open File in Excel

PowerShell provides the ability to open files directly in Microsoft Excel. This is particularly useful when you need to analyze or manipulate data within a spreadsheet.
$fileUrl = "https://yoursharepointsite.com/Shared Documents/YourFile.xlsx"
$excel = New-Object -ComObject Excel.Application
$workbook = $excel.Workbooks.Open($fileUrl)
$excel.Visible = $true
Replace "https://yoursharepointsite.com/Shared Documents/YourFile.xlsx"
with the actual URL of the file you want to open.
4. Save and Close Excel Workbook

After making changes in Excel, you can save and close the workbook using the following commands:
$workbook.Save()
$workbook.Close()
$excel.Quit()
5. Open Multiple Files

PowerShell allows you to open multiple files simultaneously. This can be beneficial when you need to work with a set of related files.
$fileUrls = @(
"https://yoursharepointsite.com/Shared Documents/File1.xlsx",
"https://yoursharepointsite.com/Shared Documents/File2.xlsx",
"https://yoursharepointsite.com/Shared Documents/File3.xlsx"
)
foreach ($fileUrl in $fileUrls) {
$excel.Workbooks.Open($fileUrl) | Out-Null
}
$excel.Visible = $true
Adjust the $fileUrls
array with the URLs of the files you want to open.
6. Use Wildcards for File Names

You can use wildcards in file names to open multiple files with similar patterns. This saves you from explicitly listing each file's URL.
$fileUrls = Get-PnPFile -Url "Shared Documents" -Filter "SalesData*.xlsx"
foreach ($fileUrl in $fileUrls) {
$excel.Workbooks.Open($fileUrl.ServerRelativeUrl) | Out-Null
}
$excel.Visible = $true
The above code opens all Excel files starting with "SalesData"
in the "Shared Documents"
library.
7. Handle Large File Sizes

When working with large files, it's important to manage memory usage effectively. PowerShell provides options to handle this gracefully.
$fileUrl = "https://yoursharepointsite.com/Shared Documents/LargeFile.xlsx"
$excel = New-Object -ComObject Excel.Application
$workbook = $excel.Workbooks.Open($fileUrl, $true, $true, $true)
$excel.Visible = $true
The additional parameters $true
in the Open
method enable read-only access and prevent updating links.
8. Open Files in Read-Only Mode

To prevent accidental modifications, you can open files in read-only mode. This is especially useful when multiple users need to access the same file simultaneously.
$fileUrl = "https://yoursharepointsite.com/Shared Documents/ReadOnlyFile.xlsx"
$excel = New-Object -ComObject Excel.Application
$workbook = $excel.Workbooks.Open($fileUrl, $true)
$excel.Visible = $true
9. Check File Existence

Before attempting to open a file, it's a good practice to check if the file exists on SharePoint. This helps prevent errors and unexpected behavior.
$fileUrl = "https://yoursharepointsite.com/Shared Documents/YourFile.xlsx"
$fileExists = Test-Path -Path $fileUrl
if ($fileExists) {
# Proceed with opening the file
} else {
Write-Host "File not found."
}
10. Open Files with Specific Extensions
You can filter files based on their extensions to open only specific types of files, such as Excel, Word, or PowerPoint.
$fileUrls = Get-PnPFile -Url "Shared Documents" -Filter "*.xlsx"
foreach ($fileUrl in $fileUrls) {
$excel.Workbooks.Open($fileUrl.ServerRelativeUrl) | Out-Null
}
$excel.Visible = $true
11. Automate File Opening
PowerShell's scheduling capabilities allow you to automate the process of opening files at specific intervals. This can be useful for regular data analysis or reporting tasks.
$fileUrl = "https://yoursharepointsite.com/Shared Documents/DailyReport.xlsx"
$excel = New-Object -ComObject Excel.Application
$workbook = $excel.Workbooks.Open($fileUrl)
$excel.Visible = $true
$schedule = New-ScheduledTaskAction -Execute "C:\Windows\System32\WindowsPowerShell\v1.0\powershell.exe" -Argument "-File 'C:\Path\To\YourScript.ps1'"
$trigger = New-ScheduledTaskTrigger -Daily -At 9AM
Register-ScheduledTask -TaskName "OpenDailyReport" -Action $schedule -Trigger $trigger
12. Open Files Based on Last Modified Date
If you want to open files based on their last modification date, you can use the Get-PnPFile
cmdlet with the -Filter
parameter.
$lastModifiedDate = (Get-Date).AddDays(-7)
$fileUrls = Get-PnPFile -Url "Shared Documents" -Filter "LastModifiedDate ge $lastModifiedDate"
foreach ($fileUrl in $fileUrls) {
$excel.Workbooks.Open($fileUrl.ServerRelativeUrl) | Out-Null
}
$excel.Visible = $true
13. Open Files in Different Workbooks
By default, PowerShell opens files in the same Excel instance. However, you can specify to open each file in a new workbook if needed.
$fileUrls = @(
"https://yoursharepointsite.com/Shared Documents/File1.xlsx",
"https://yoursharepointsite.com/Shared Documents/File2.xlsx"
)
foreach ($fileUrl in $fileUrls) {
$workbook = $excel.Workbooks.Add()
$workbook.Worksheets.Item(1).Name = Split-Path -Path $fileUrl -Leaf
$workbook.Worksheets.Item(1).Activate()
$workbook.Worksheets.Item(1).Range("A1").Value = "Data from " + $fileUrl
$workbook.Worksheets.Item(1).Range("A1").Font.Bold = $true
}
$excel.Visible = $true
14. Handle Errors Gracefully
When opening files, it's important to handle potential errors gracefully. You can use try-catch blocks to catch and handle exceptions.
try {
$fileUrl = "https://yoursharepointsite.com/Shared Documents/YourFile.xlsx"
$excel = New-Object -ComObject Excel.Application
$workbook = $excel.Workbooks.Open($fileUrl)
$excel.Visible = $true
} catch {
Write-Host "An error occurred: $($_.Exception.Message)"
}
15. Retrieve File Metadata
Before opening a file, you might want to retrieve its metadata, such as the author, creation date, or modification date. This information can be useful for various purposes.
$fileUrl = "https://yoursharepointsite.com/Shared Documents/YourFile.xlsx"
$file = Get-PnPFile -Url $fileUrl
Write-Host "File Name: $($file.Name)"
Write-Host "Author: $($file.Author)"
Write-Host "Created: $($file.Created)"
Write-Host "Modified: $($file.Modified)"
16. Open Files in Specific Folders
You can restrict the file opening process to specific folders on SharePoint. This helps organize and manage files more efficiently.
$folderUrl = "https://yoursharepointsite.com/Shared Documents/Finance"
$files = Get-PnPFile -Url $folderUrl
foreach ($file in $files) {
Write-Host $file.Name
}
17. Open Files with Specific Keywords
If you have a large number of files, you can search for files containing specific keywords in their names. This helps locate relevant files quickly.
$keyword = "Report"
$fileUrls = Get-PnPFile -Url "Shared Documents" -Filter "Contains(Name, '$keyword')"
foreach ($fileUrl in $fileUrls) {
$excel.Workbooks.Open($fileUrl.ServerRelativeUrl) | Out-Null
}
$excel.Visible = $true
18. Open Files with Advanced Filtering
PowerShell's filtering capabilities allow you to apply advanced filters to retrieve specific files. This is particularly useful when dealing with complex file structures.
$fileUrls = Get-PnPFile -Url "Shared Documents" -Filter "startswith(Name, 'Sales') and endsWith(Name, '.xlsx')"
foreach ($fileUrl in $fileUrls) {
$excel.Workbooks.Open($fileUrl.ServerRelativeUrl) | Out-Null
}
$excel.Visible = $true
19. Open Files with Specific Authors
If you want to open files created or modified by specific authors, you can use the Author
property in the filter.
$author = "John Doe"
$fileUrls = Get-PnPFile -Url "Shared Documents" -Filter "Author eq '$author'"
foreach ($fileUrl in $fileUrls) {
$excel.Workbooks.Open($fileUrl.ServerRelativeUrl) | Out-Null
}
$excel.Visible = $true
20. Open Files with Custom Properties
SharePoint allows you to define custom properties for files. You can use these properties to filter and open files based on specific criteria.
$customProperty = "Project"
$fileUrls = Get-PnPFile -Url "Shared Documents" -Filter "Project eq 'ProjectX'"
foreach ($fileUrl in $fileUrls) {
$excel.Workbooks.Open($fileUrl.ServerRelativeUrl) | Out-Null
}
$excel.Visible = $true
21. Open Files with Version Control
If your SharePoint site has version control enabled, you can specify the version of the file you want to open. This ensures you're working with the correct version of the file.
$fileUrl = "https://yoursharepointsite.com/Shared Documents/YourFile.xlsx"
$version = 2
$excel = New-Object -ComObject Excel.Application
$workbook = $excel.Workbooks.Open($fileUrl, $false, $false, $version)
$excel.Visible = $true
22. Open Files with Custom Columns
SharePoint lists can have custom columns. You can use these columns to filter and open files based on their values.
$customColumn = "Status"
$fileUrls = Get-PnPFile -Url "Shared Documents" -Filter "Status eq 'Approved'"
foreach ($fileUrl in $fileUrls) {
$excel.Workbooks.Open($fileUrl.ServerRelativeUrl) | Out-Null
}
$excel.Visible = $true
23. Open Files with Advanced Sorting
You can sort files based on their properties, such as name, size, or modification date, to organize them before opening.
$fileUrls = Get-PnPFile -Url "Shared Documents" -SortBy "Modified, Descending"
foreach ($fileUrl in $fileUrls) {
$excel.Workbooks.Open($fileUrl.ServerRelativeUrl) | Out-Null
}
$excel.Visible = $true
24. Open Files with Batch Processing
PowerShell's batch processing capabilities allow you to open multiple files in parallel, improving performance for large datasets.
$fileUrls = Get-PnPFile -Url "Shared Documents"
$jobs = @()
foreach ($fileUrl in $fileUrls) {
$job = Start-Job -ScriptBlock {
param($fileUrl)
$excel = New-Object -ComObject Excel.Application
$workbook = $excel.Workbooks.Open($fileUrl)
$excel.Visible = $true
} -ArgumentList $fileUrl.ServerRelativeUrl
$jobs += $job
}
Wait-Job -Job $jobs | Receive-Job
25. Open Files with Custom Scripts
You can create custom scripts or functions to handle specific file opening scenarios. This allows for more complex logic and customization.
function OpenExcelFile($fileUrl) {
$excel = New-Object -ComObject Excel.Application
$workbook = $excel.Workbooks.Open($fileUrl)
$excel.Visible = $true
}
$fileUrl = "https://yoursharepointsite.com/Shared Documents/YourFile.xlsx"
OpenExcelFile -fileUrl $fileUrl
Conclusion
PowerShell offers a wide range of capabilities to streamline the process of opening files on SharePoint. By utilizing these tips and techniques, you can enhance your productivity and efficiently manage files within your SharePoint environment. Whether you’re opening files in Excel, filtering based on specific criteria, or automating the process, PowerShell provides the tools to master the